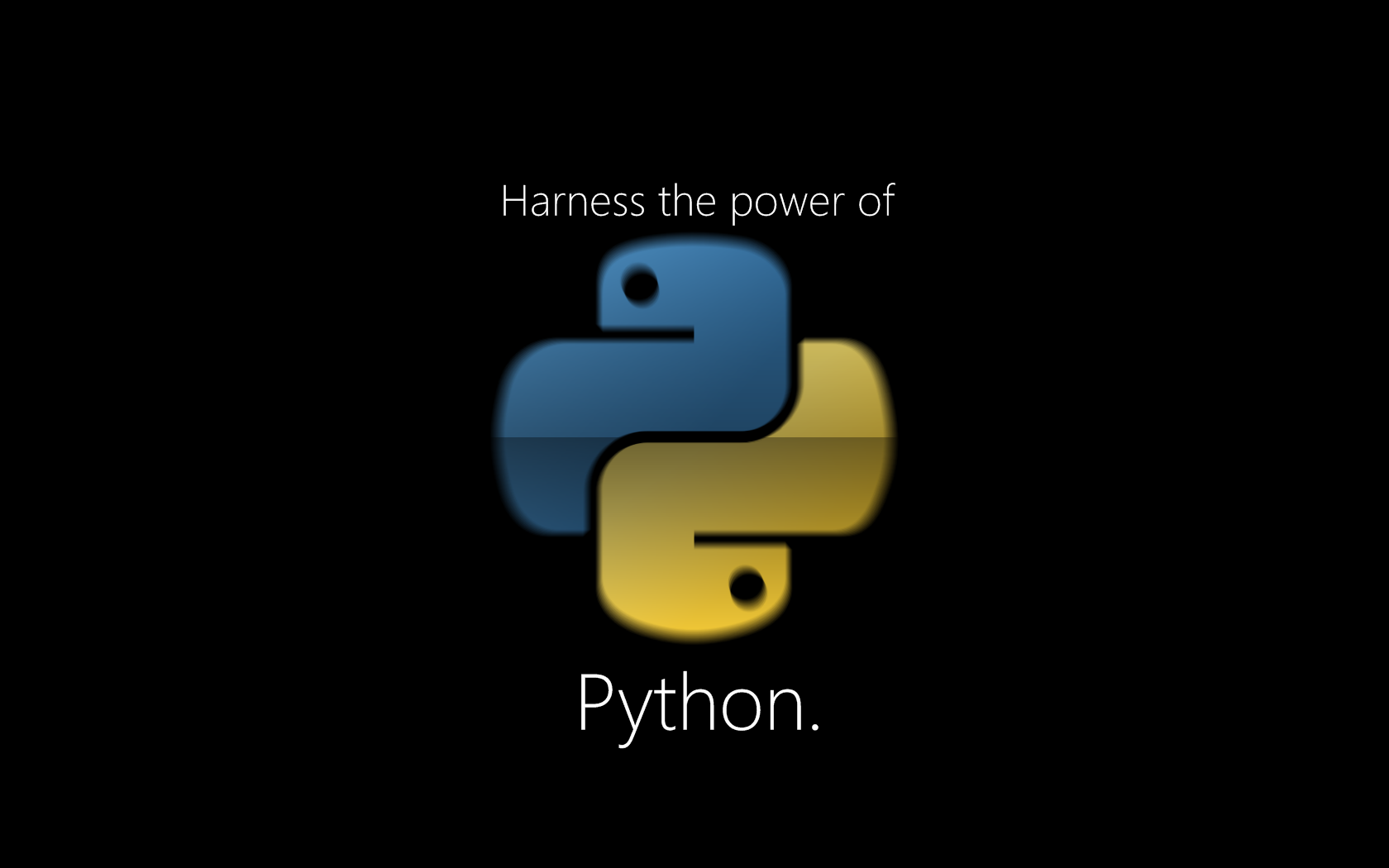
Dictionary is a mapping data structure containing a set of elements where each element is a key-value pair. Dictionaries in other programming languages are called as Hashes or Associative arrays. Dictionaries are indexed by keys rather than integer numbers like in lists or tuples. An example of a simple dictionary is as follows.

Alternative Ways to Create a Dictionary
We can also create a dictionary using the dict constructor function. The dict() function creates a dictionary directly from a list of key-value pairs. Another way is to create a dictionary using keyword arguments where keys must be specified as strings. Both of these mechanisms are shown as follows.
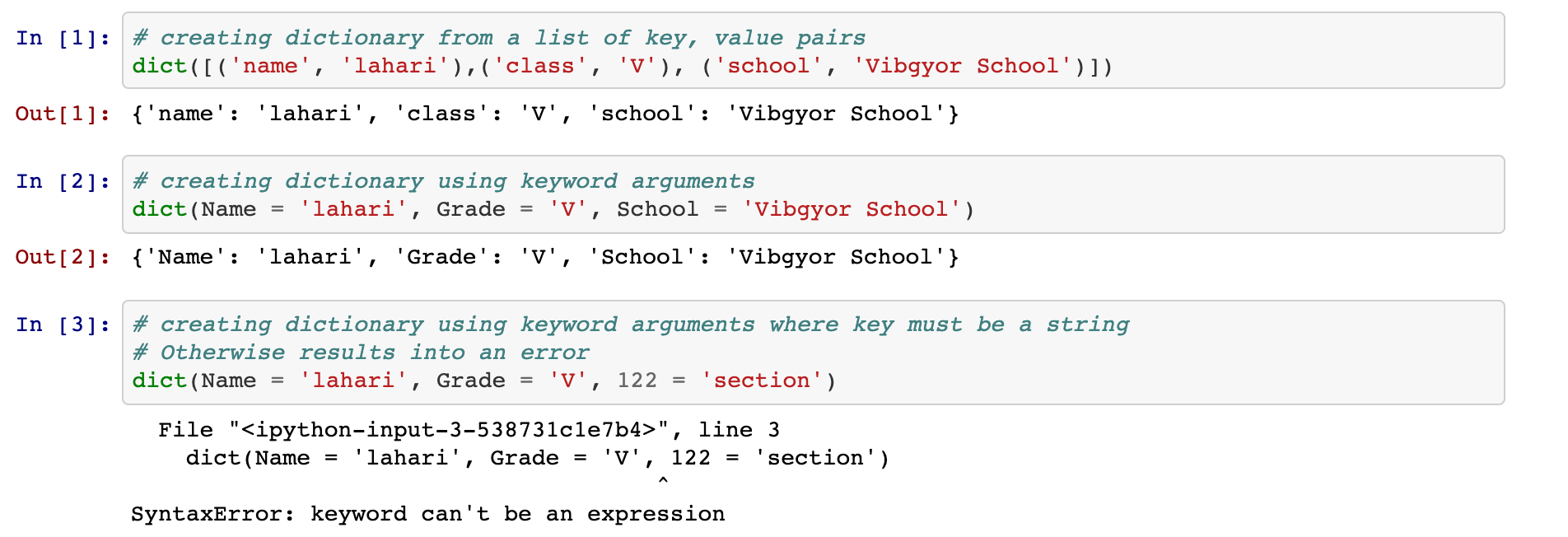
Adding Elements to a Dictionary
Adding elements to the dictionary is quite simple and does not need any function such as append like in lists. Assigning a value to a non-existing key automatically adds an element to it. If the key already exists it overwrites the new value given. Following is an example demonstrating the same.
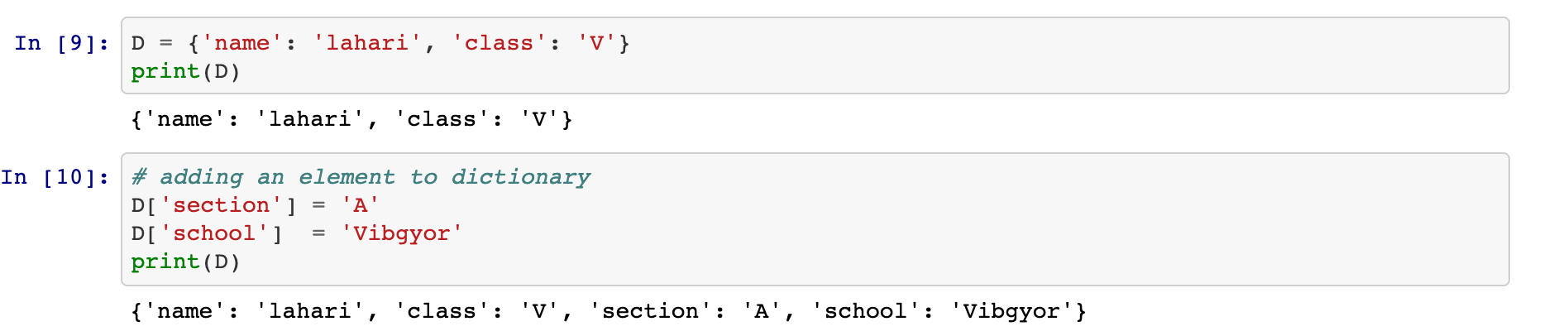
How Elements in the Dictionary are Accessed?
Elements in a dictionary can be accessed if we have a key to retrieve the value whereas the reverse is not true. If we try to access a key using the value it will result in a key error. Following is an example demonstrating the same.
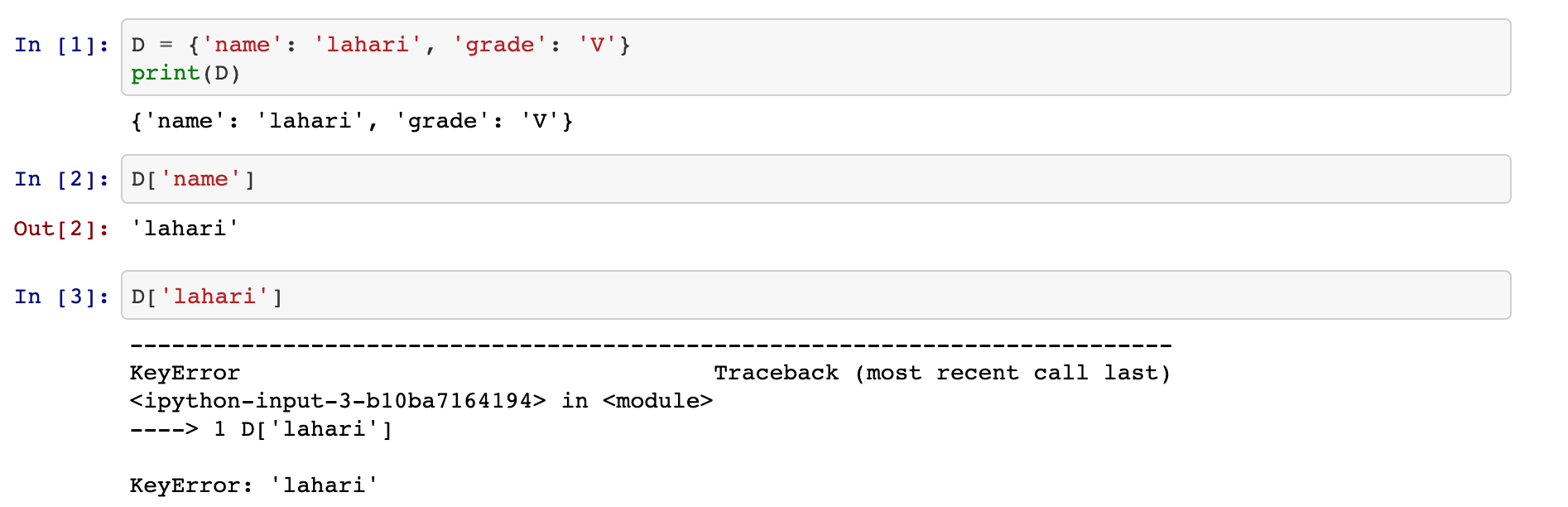
Are Keys Unique?
Keys in a dictionary must be unique otherwise the latest value of a key will overwrite the old value when you update the same key. Values need not be unique and are possible even to have duplicate values. Following is an example depicting the same.
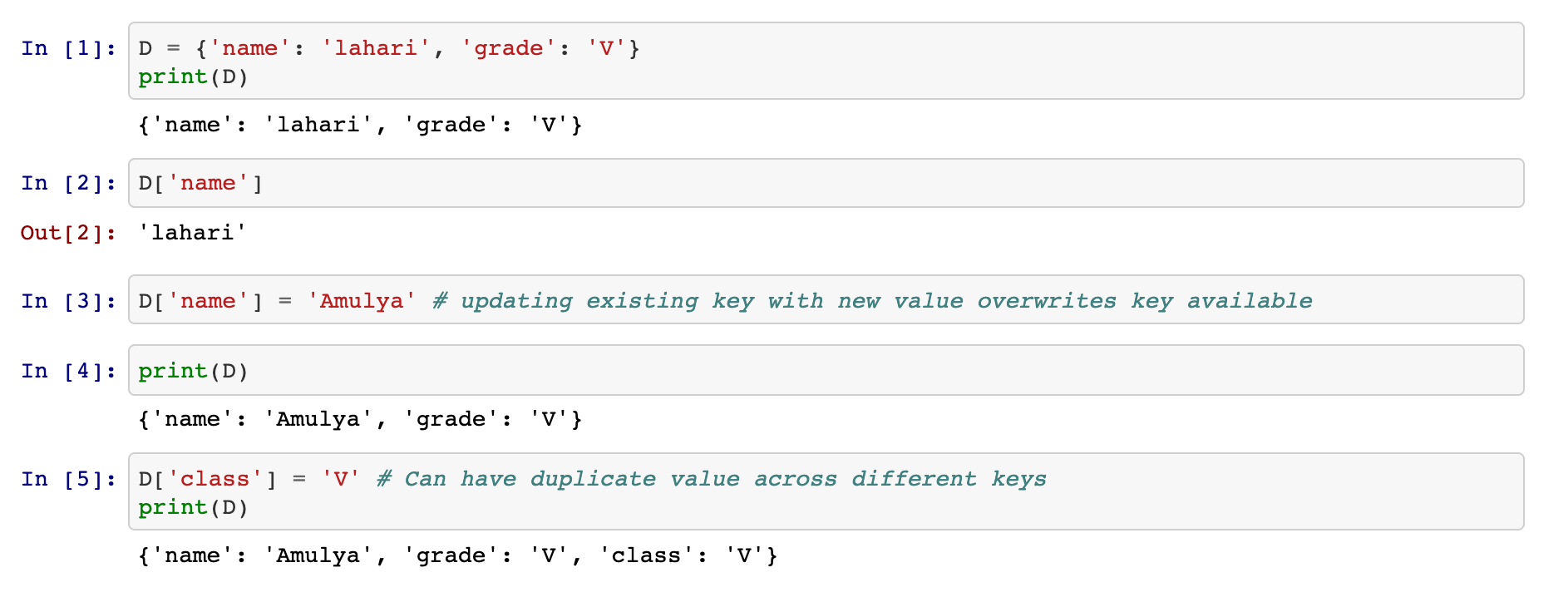
What Object Type could be a Key?
While we can have any object as the value of a dictionary whereas there are certain restrictions on the objects to be used as Key. Keys in the dictionary must be an immutable object data type. That means any object which cannot change its value once it is created can be used as a key.
Some of the immutable objects in python are integer, float, complex, string, bool, tuple, range operator, frozen sets, Unicode, etc. All these objects once created cannot be modified and hence can be used as a key in dictionaries. The following are some of the examples.
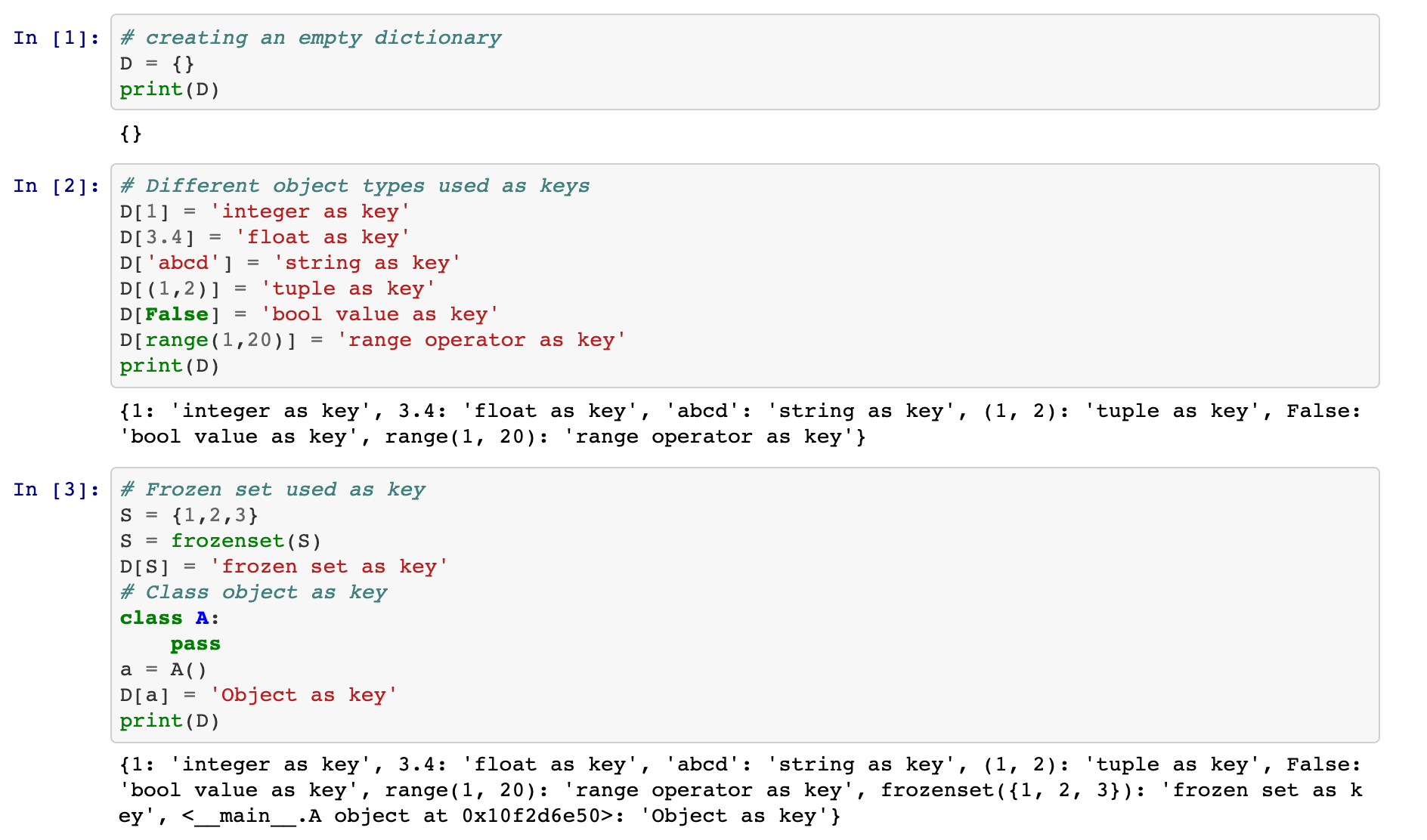
In the above example Object is used as a key because it is immutable and the object reference address does not change after its creation.
For deep dive on Python internals follow me on Linkedin –https://www.linkedin.com/in/krishnabvv/